Streamlit Tutorial: Dynamic Confirmation Modals & Session State
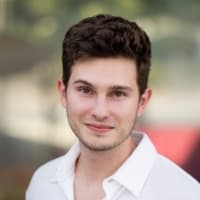
Senior Software Engineer
We recently turned to Streamlit to rapidly prototype an LLM-powered application for a client in the EdTech space. While it may require a shift in thinking for those more familiar with React and frontend Javascript frameworks, Streamlit provides a powerful tool for creating a dynamic UX from a simple Python script, and we use it often. In this particular case we needed to include a dynamic confirmation modal to improve the user experience. Streamlit doesn’t provide that capability out of the box; here’s how we built it ourselves.
Today, we’re going to embark on an exciting journey into the world of Streamlit, focusing on creating dynamic, user-interactive confirmation modals. This tutorial is designed for those who have dabbled in Streamlit and are looking to expand their skill set with more advanced features. So, let’s dive in and add some interactive flair to your Streamlit apps!
Streamlit’s Execution Model: A Quick Refresher
Before we jump into the code, it’s crucial to understand Streamlit’s unique execution model. Streamlit apps are stateless by default. This means that each time a user interacts with the app, the entire script reruns from the top. While this makes Streamlit apps straightforward to write, it poses a challenge for maintaining state across runs. That’s where the session state comes into play.
The Power of Session State
Session state in Streamlit is like a persistent memory that retains information across runs, allowing you to store user inputs, variables, and other data. It’s a game-changer for creating more complex, interactive apps.
Setting Up the Modal’s Session State
Our first step is to initialize the session state for our confirmation modal. We’ll set a flag in the session state that dictates whether the modal should be shown. Here’s how it’s done:
if st.session_state.get("show_modal"):
# Code to display the modal will go here
This snippet checks if the "show_modal"
key is set in the session state. If it’s True
, we’ll proceed to render the modal.
Designing the Confirmation Modal
Streamlit allows us to leverage HTML and CSS for custom UI elements, giving us the freedom to create sophisticated and visually appealing components.
Crafting the Modal with CSS
Let’s explore the CSS used for our modal:
- Positioning: We’re using
position: fixed
andtransform: translate(-50%, -50%)
to center our modal on the screen. - Visual Appeal: A white background, rounded corners, and padding give our modal a clean and modern look.
- Layering with Z-index: The modal has a z-index of 9999, placing it above other page elements.
Implementing the Modal in Streamlit
To create the modal, we use st.markdown
and inject our CSS styles to create a dimmed background effect:
modal_background_style = {
# CSS styles for the dimmed background
}
st.markdown(
f"""
<div id="modal_background" style="{get_element_style(modal_background_style)}"></div>
""",
unsafe_allow_html=True,
)
The unsafe_allow_html=True
parameter is essential for injecting raw HTML and CSS. While powerful, it should be used judiciously to avoid security risks.
Building the Confirmation Dialog
Inside the modal, we include the confirmation message and action buttons using Streamlit’s layout options:
with st_stylable_container(...):
# Code for confirmation message and action buttons
Here, users are presented with a clear message and options to either cancel or confirm their action.
Triggering the Modal
In our app, we have a button that, when clicked, sets the show_modal
session state. This action triggers the display of the modal.
if st.button("Activate Modal"):
st.session_state["show_modal"] = True
Deep Dive: The Full Implementation
Let’s consolidate our learning and walk through the entire implementation, from setting up the session state to creating and displaying the modal.
Setting the Stage: Initializing Session State
We begin by checking the session state to determine if the modal should be displayed.
if st.session_state.get("show_modal"):
# This is where our modal code will be placed
The Art of CSS in Streamlit
Next, we define the CSS for our modal, focusing on aesthetics and functionality.
modal_background_style = {
# Detailed CSS for the modal background
}
st.markdown(...)
with st_stylable_container(...):
# CSS and HTML for the modal's content
User Interaction: Displaying the Modal
We now set up the user interaction that triggers the modal. This is where the magic happens!
if st.button("Show Modal"):
st.session_state["show_modal"] = True
Wrapping Up: The Importance of Styling and Interactivity
Through this tutorial, you’ve learned how to effectively use session state and CSS to create dynamic, interactive modals in Streamlit. These modals not only enhance the functionality of your apps but also significantly improve the user experience.
Your Turn to Shine
Now it’s over to you! Experiment with different styles, tweak the modal’s logic, and explore how these elements can transform your Streamlit applications into more engaging and interactive experiences.
Remember, the Streamlit community is an excellent resource for inspiration and support. Share your creations, learn from others, and continue to grow as a Streamlit developer.
And if you need help implementing a streamlit app for your project, send us a message or schedule a call — we look forward to learning about your work and exploring how we can help!
Let Aprime help you overcome your challenges
and build your core technology
Are you ready to accelerate?